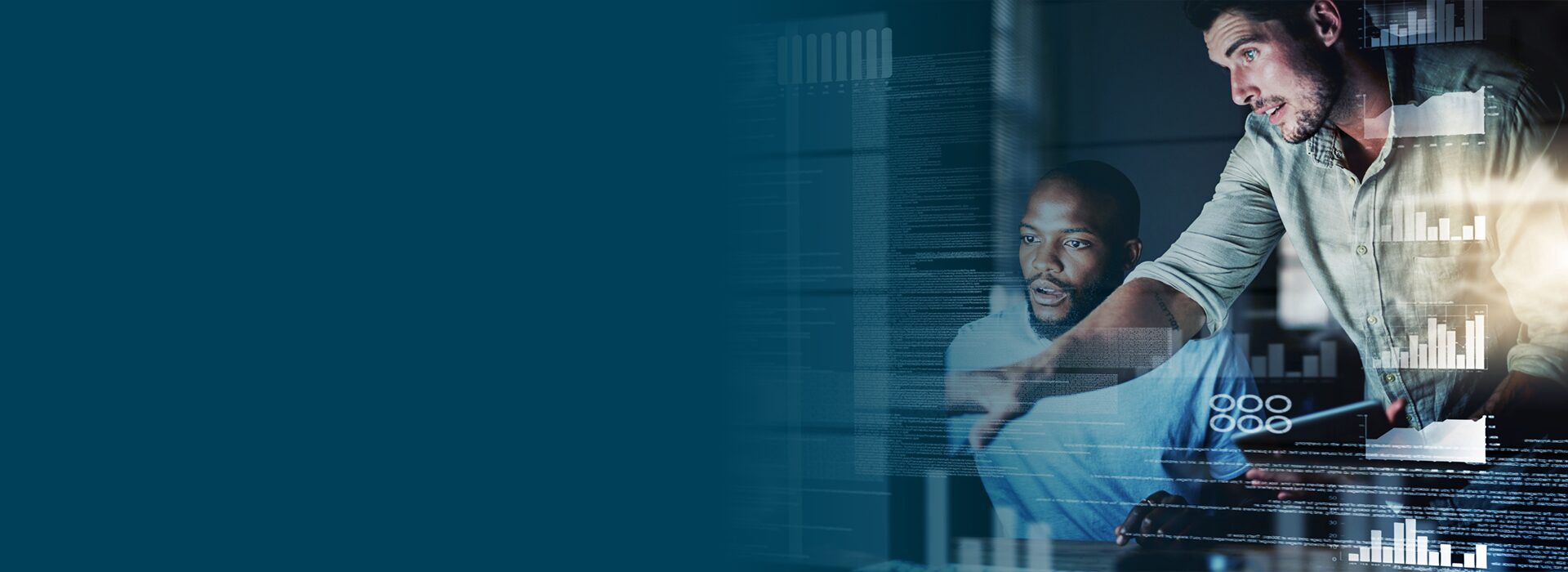
Professional IAM Services from the Leading Identity and Access Management Provider:
Consulting, System Integration, Managed Services
iC Consult is your expert Identity & Access Management (IAM) partner, offering over 25 years of industry-leading expertise. With a global team of 850+ professionals and a strong network of top-tier partners, we provide scalable, world-class IAM solutions. Trusted by renowned brands worldwide, we are dedicated to driving your IAM success and innovation.
25+
Years Experience
850+
Employees
30+
Partners
300+
Active Customers
20+
Global Offices
Vendor independent
Premium level delivery partnerships
Partner & analyst awarded
Our IAM Services for the Entire Identity and Access Management Lifecycle
IAM Consulting and Advisory
IAM Consulting and Advisory
With over 25 years of experience in Identity and Access Management, our team stands ready to guide you through every stage of your IAM journey. From understanding your current state to developing your unique roadmap, our holistic approach ensures you are well-prepared for success – whether you are just starting out or looking to optimize your existing IAM strategy.
IAM Architecture, Integration and Implementation
IAM Architecture, Integration and Implementation
With iC Consult, your IAM vision becomes a reality. Our experts design, implement and integrate solutions that align perfectly with your business needs. Experience a future ready IAM infrastructure that is secure, efficient, and customized to your individual needs.
IAM Support, Maintenance and Operation
IAM Support, Maintenance and Operation
Entrust your IAM infrastructure to experts who prioritize security and efficiency. Our specialized 2nd and 3rd level support ensures your IAM systems run smoothly and are maintained on a regular basis. Benefit from automated service monitoring, an efficient ticket system, customizable support models, and remote, international support from key locations.
IAM Managed Services
IAM Managed Services
Experience IAM like never before with iC Consult’s IAM Managed Services. We are your partner from start to finish, delivering tailored solutions that adapt to your business. Our end-to-end services range from advisory to support and operations. You have full flexibility to configure and scale your solutions according to your needs, and a dedicated service delivery manager always at your side.
Our Valued Customers Speak for Us:
IAM Topics: Where Expertise Meets Innovation
iC Consult is your trusted IAM partner, excelling in critical IAM topics. From complex challenges to specific requirements, we provide expertise in every aspect of IAM.
Access Management & Federation
Integrate and manage user access across platforms.
IAM Cloud Migration
Transition to cloud-based IAM for flexibility and scalability.
Customer Identity & Access Management
Deliver secure, seamless, and personalized customer experiences.
Identity Centric Security
Place identity at the core of your security strategy.
Identity Governance & Administration
Efficiently manage digital identities, access rights, and more.
Privileged Access Management
Safeguard critical systems and data.
Zero Trust
Verify every access request to ensure maximum security.
Connect with our Experts
Whether you have questions, need advice, or are ready to get started with Identity and Access Management,
our dedicated team is here to assist every step of the way.
Our Partner Network
Collaborating for Your IAM Success
At iC Consult, we believe in providing the best and most comprehensive IAM solutions possible. Our partner network, featuring over 30 top-tier
IAM product vendors, ensures you receive unbiased, holistic solutions tailored to your specific needs.
IAM-Related Content that Inspires
Valuable Insights and Expert Guidance to Drive your IAM Strategy
KuppingerCole Leadership Compass 2023: IAM System Integrators
Download Report
Why Organizations Should Take Action Now to Prepare for NIS2
Read BlogpostMastering Global IAM: From Zero Trust Principles to ITDR
Watch WebinarMeet us in Person
Industry, Partner, and iC Consult Hosted Events
Results: 19
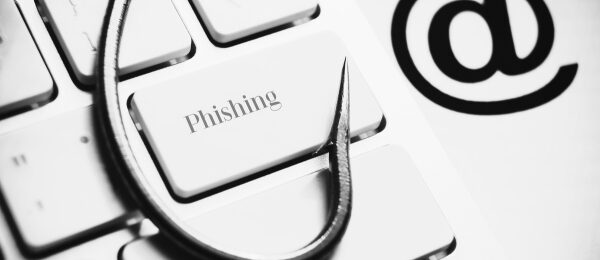
Event
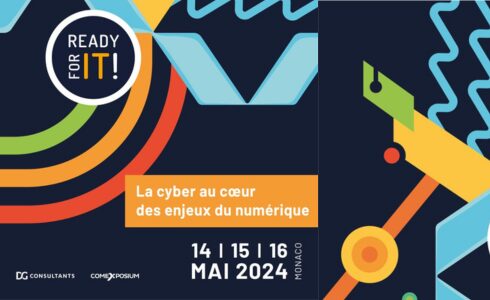